[SOLVED]-REACTJS CALLING TWO FUNCTIONS WITH ONCLICK-REACTJS
REACTJS CALLING TWO FUNCTIONS WITH ONCLICK-REACTJS
as suggested in one of the answers, use function binding or just opt for arrow function:
<li
key={index}
onclick={(event)=>this.onbuttonclick(event, index)}
style={{ color: this.state.colour }}
>
changecolour = (i) => {
console.log(i);
this.setstate({
colour: 'grey',
});
};
crossline = (event) => {
const element = event.target;
element.classlist.toggle('crossed-line');
};
onbuttonclick=(event, index)=> {
this.changecolour(index);
this.crossline(event);
}
full example based on question description(more details would have been even helpful :d ):
outpu:-
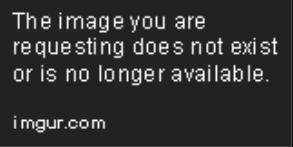
source code:
import react, { component } from "react";
import "./style.css";
const list = ["one", "two", "three", "four", "five"];
export default class app extends component {
constructor(props) {
super(props);
this.state = {
colour: "black"
};
}
changecolour = i => {
console.log(i);
this.setstate({
colour: "grey",
index: i
});
};
crossline = event => {
const element = event.target;
console.log(element);
element.classlist.toggle("crossed-line");
};
onbuttonclick = (event, index) => {
this.changecolour(index);
this.crossline(event);
};
render() {
return (
<div>
<ul>
{list.map((elem, index) => (
<li
classname={index == this.state.index ? "crossed-line" : null}
key={index}
onclick={event => this.onbuttonclick(event, index)}
style={{
color: index == this.state.index ? this.state.colour : "black"
}}
>
{elem}
</li>
))}
</ul>
</div>
);
}
}
css:-
h1,
p {
font-family: lato;
}
.crossed-line {
text-decoration: line-through;
}
first: you must bind the function inside the constructor, something like this:
constructor() {
super();
this.onbuttonclick = this.onbuttonclick.bind(this);
}
second: you need to pass the event and the index to the onbuttonclick function (i recommend you use arrow function):
<li
key={index}
onclick={(event) => this.onbuttonclick(event, index)}
style={{ color: this.state.colour }}
>
with this, the function onbuttonclick can receive the event and thus pass it to the function crossline, leaving the code similar to this:
changecolour = (index) => {
console.log(index);
this.setstate({
colour: 'grey',
});
};
crossline = (event) => {
const element = event.target;
element.classlist.toggle('crossed-line');
};
onbuttonclick=(event, index)=> {
this.changecolour(index);
this.crossline(event);
}
you should define your onclick
as an arrow function. otherwise, this
is undefined unless you bind
it to the current context. also, not related to the undefined
issue but you should pass params to the function calls.