FIND OBJECT BY ID IN AN ARRAY OF JAVASCRIPT OBJECTS
FIND OBJECT BY ID IN AN ARRAY OF JAVASCRIPT OBJECTS

var customerarray =
[
{ 'id': '1', 'name': 'Shelley Burke', 'address': 'P.O. Box 78934', 'country': 'Norway' },
{ 'id': '2', 'name': 'Regina Murphy', 'address': 'Calle del Rosal 4', 'country': 'Australia' },
{ 'id': '3', 'name': 'Ian Devling', 'address': 'Av. das Americanas 12.890', 'country': 'Sweden' },
{ 'id': '4', 'name': 'Peter Wilson', 'address': 'Tiergartenstraße 5', 'country': 'Germany' },
{ 'id': '5', 'name': 'Sven Petersen', 'address': 'Frahmredder 112a', 'country': 'UK' },
{ 'id': '6', 'name': 'Hatlevegen 5', 'address': '3400 - 8th Avenue Suite 210', 'country': 'USA' },
{ 'id': '7', 'name': 'Marry 5', 'address': 'Brovallavägen ', 'country': 'Sweden' },
]
As you can see above array, we have an array of the customer object which has id, name, address, and country property. Now let’s say, I want to be able to search customerarrayobj for an object with an “id” matching for example with id=”2″.

Find object by id in an array of JavaScript objects
<script type="text/javascript">
//I think the easiest way would be the following, but it won't work on Internet Explorer 8 (or earlier):
var customerarrayobj =
[
{ 'id': '1', 'name': 'Shelley Burke', 'address': 'P.O. Box 78934', 'country': 'Norway' },
{ 'id': '2', 'name': 'Regina Murphy', 'address': 'Calle del Rosal 4', 'country': 'Australia' },
{ 'id': '3', 'name': 'Ian Devling', 'address': 'Av. das Americanas 12.890', 'country': 'Sweden' },
{ 'id': '4', 'name': 'Peter Wilson', 'address': 'Tiergartenstraße 5', 'country': 'Germany' },
{ 'id': '5', 'name': 'Sven Petersen', 'address': 'Frahmredder 112a', 'country': 'UK' },
{ 'id': '6', 'name': 'Hatlevegen 5', 'address': '3400 - 8th Avenue Suite 210', 'country': 'USA' },
{ 'id': '7', 'name': 'Marry 5', 'address': 'Brovallavägen ', 'country': 'Sweden' },
]
const result = customerarrayobj.find(x => x.id === '2');
if (result)//check if result is not undefined in case if record not find the array object
{
document.write("Result found in the array with id:" + result.id +
"<br><br> customer name:" + result.name +
" <br>address:" + result.address +
"<br>country:" + result.country+"<br>");
}
</script>
once that object is found in the array, we get the information from the object and write it in the document.
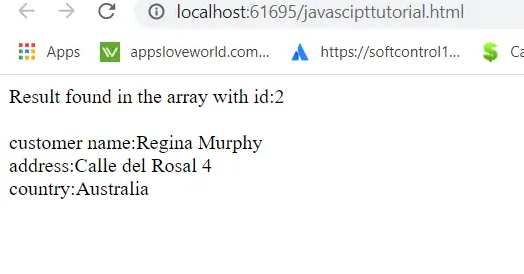
Find a value in an array of objects in Javascript
Let’s take a real-time example for finding a value in an array of objects in Javascript. I have created an HTML page in which I have a text input in which the user can input the id. and based on that id, I’m going to search for the object by its id, and then I will show that object in the div.
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<input type="number" id="txtinput" onchange="Filterarray()"/>
<div id="divresult"></div>
<script type="text/javascript">
//I think the easiest way would be the following, but it won't work on Internet Explorer 8 (or earlier):
var customerarrayobj =
[
{ 'id': '1', 'name': 'Shelley Burke', 'address': 'P.O. Box 78934', 'country': 'Norway' },
{ 'id': '2', 'name': 'Regina Murphy', 'address': 'Calle del Rosal 4', 'country': 'Australia' },
{ 'id': '3', 'name': 'Ian Devling', 'address': 'Av. das Americanas 12.890', 'country': 'Sweden' },
{ 'id': '4', 'name': 'Peter Wilson', 'address': 'Tiergartenstraße 5', 'country': 'Germany' },
{ 'id': '5', 'name': 'Sven Petersen', 'address': 'Frahmredder 112a', 'country': 'UK' },
{ 'id': '6', 'name': 'Hatlevegen 5', 'address': '3400 - 8th Avenue Suite 210', 'country': 'USA' },
{ 'id': '7', 'name': 'Marry 5', 'address': 'Brovallavägen ', 'country': 'Sweden' },
]
function Filterarray()
{
var id = document.getElementById("txtinput").value;
if (id)
{
const result = customerarrayobj.find(x => x.id === id);
if (result)//check if result is not undefined in case if record not find the array object
{
document.getElementById("divresult").innerHTML = "Result found in the array with id:" + result.id +
"<br><br> customer name:" + result.name +
" <br>address:" + result.address +
"<br>country:" + result.country + "<br>";
}
}
}
</script>
</body>
</html>
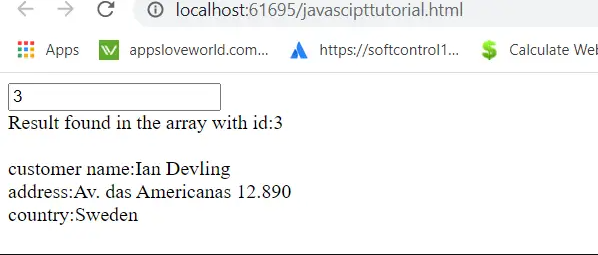