DISPLAY JSON DATA IN HTML TABLE USING JAVASCRIPT DYNAMICALLY
JSON DATA IN HTML TABLE USING JAVASCRIPT DYNAMICALLY

var employess = [
{
"EmployeeID": "1",
"EmployeeName": "Hanari Carnes",
"Address": "Rua do Paço, 67",
"City": "Rio de Janeiro",
"Country": "Brazil"
},
{
"EmployeeID": "2",
"EmployeeName": "Wolski",
"Address": "ul. Filtrowa 68",
"City": "Walla",
"Country": "Poland"
},
{
"EmployeeID": "3",
"EmployeeName": "Lehmanns Marktstand",
"Address": "Magazinweg 7",
"City": "Frankfurt a.M.",
"Country": "Germany"
}
,
{
"EmployeeID": "4",
"EmployeeName": "Let's Stop N Shop",
"Address": "87 Polk St. Suite 5",
"City": "San Francisco",
"Country": "USA"
},
{
"EmployeeID": "5",
"EmployeeName": "Lazy K Kountry Store",
"Address": "12 Orchestra Terrace",
"City": "Walla Walla",
"Country": "USA"
}
,
{
"EmployeeID": "6",
"EmployeeName": "Island Trading",
"Address": "Garden House Crowther Way",
"City": "Cowes",
"Country": "UK"
}
]
Now I want to generate a table from this employee JSON using Javascript.
Convert json array data to a html table
<!DOCTYPE html>
<html>
<head>
<title> Use of JQuery to Add Edit Delete Table Row </title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" />
</head>
<body>
<div class="container">
<h1>Employee List</h1>
<br />
<div id="employeedivcontainer">
</div>
</div>
</body>
</html>
<script type="text/javascript">
var employess = [
{
"EmployeeID": "1",
"EmployeeName": "Hanari Carnes",
"Address": "Rua do Paço, 67",
"City": "Rio de Janeiro",
"Country": "Brazil"
},
{
"EmployeeID": "2",
"EmployeeName": "Wolski",
"Address": "ul. Filtrowa 68",
"City": "Walla",
"Country": "Poland"
},
{
"EmployeeID": "3",
"EmployeeName": "Lehmanns Marktstand",
"Address": "Magazinweg 7",
"City": "Frankfurt a.M.",
"Country": "Germany"
}
,
{
"EmployeeID": "4",
"EmployeeName": "Let's Stop N Shop",
"Address": "87 Polk St. Suite 5",
"City": "San Francisco",
"Country": "USA"
},
{
"EmployeeID": "5",
"EmployeeName": "Lazy K Kountry Store",
"Address": "12 Orchestra Terrace",
"City": "Walla Walla",
"Country": "USA"
}
,
{
"EmployeeID": "6",
"EmployeeName": "Island Trading",
"Address": "Garden House Crowther Way",
"City": "Cowes",
"Country": "UK"
}
]
convertJsontoHtmlTable();
function convertJsontoHtmlTable()
{
//Getting value for table header
// {'EmployeeID', 'EmployeeName', 'Address' , 'City','Country'}
var tablecolumns = [];
for (var i = 0; i < employess.length; i++) {
for (var key in employess[i]) {
if (tablecolumns.indexOf(key) === -1) {
tablecolumns.push(key);
}
}
}
//Creating html table and adding class to it
var tableemployee = document.createElement("table");
tableemployee.classList.add("table");
tableemployee.classList.add("table-striped");
tableemployee.classList.add("table-bordered");
tableemployee.classList.add("table-hover")
//Creating header of the HTML table using
//tr
var tr = tableemployee.insertRow(-1);
for (var i = 0; i < tablecolumns.length; i++) {
//header
var th = document.createElement("th");
th.innerHTML = tablecolumns[i];
tr.appendChild(th);
}
// Add employee JSON data in table as tr or rows
for (var i = 0; i < employess.length; i++) {
tr = tableemployee.insertRow(-1);
for (var j = 0; j < tablecolumns.length; j++) {
var tabCell = tr.insertCell(-1);
tabCell.innerHTML = employess[i][tablecolumns[j]];
}
}
//Final step , append html table to the container div
var employeedivcontainer = document.getElementById("employeedivcontainer");
employeedivcontainer.innerHTML = "";
employeedivcontainer.appendChild(tableemployee);
}
</script>
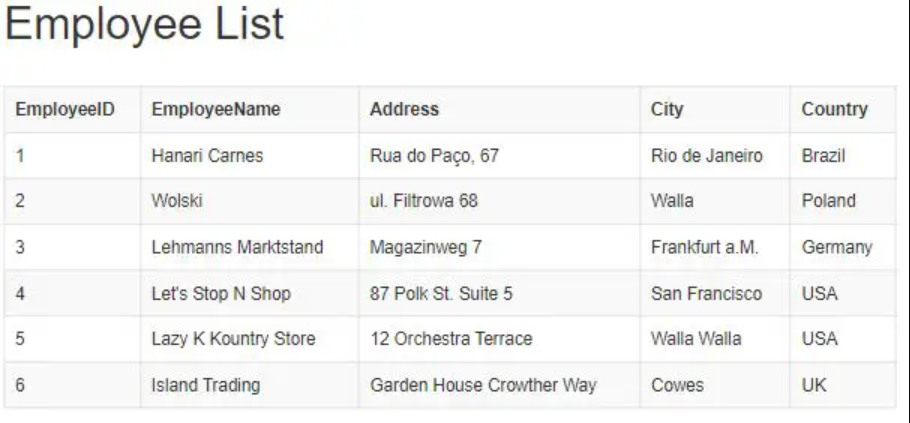
Code explanation
I’m looping through the employee array in order to extract the headers of the table.
//Getting value for table header
// {'EmployeeID', 'EmployeeName', 'Address' , 'City','Country'}
var tablecolumns = [];
for (var i = 0; i < employess.length; i++) {
for (var key in employess[i]) {
if (tablecolumns.indexOf(key) === -1) {
tablecolumns.push(key);
}
}
}
Here we are creating an HTML table element and adding class to it.
//Creating html table and adding class to it
var tableemployee = document.createElement("table");
tableemployee.classList.add("table");
tableemployee.classList.add("table-striped");
tableemployee.classList.add("table-bordered");
tableemployee.classList.add("table-hover")
we generate the header of the HTML table using
//Creating header of the HTML table using
//tr
var tr = tableemployee.insertRow(-1);
for (var i = 0; i < tablecolumns.length; i++) {
//header
var th = document.createElement("th");
th.innerHTML = tablecolumns[i];
tr.appendChild(th);
}
Adding employee JSON data in the table
//Creating header of the HTML table using
//tr
var tr = tableemployee.insertRow(-1);
for (var i = 0; i < tablecolumns.length; i++) {
//header
var th = document.createElement("th");
th.innerHTML = tablecolumns[i];
tr.appendChild(th);
}
Append HTML table to the container div
//Final step , append html table to the container div
var employeedivcontainer = document.getElementById("employeedivcontainer");
employeedivcontainer.innerHTML = "";
employeedivcontainer.appendChild(tableemployee);