HOW TO ADD, EDIT AND DELETE ROWS OF AN HTML TABLE WITH JQUERY?
ADD, EDIT AND DELETE ROWS OF AN HTML TABLE WITH JQUERY
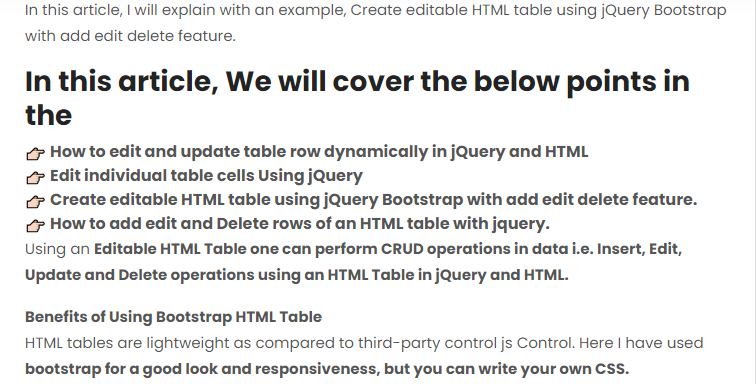
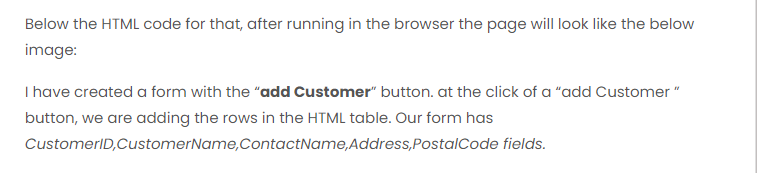
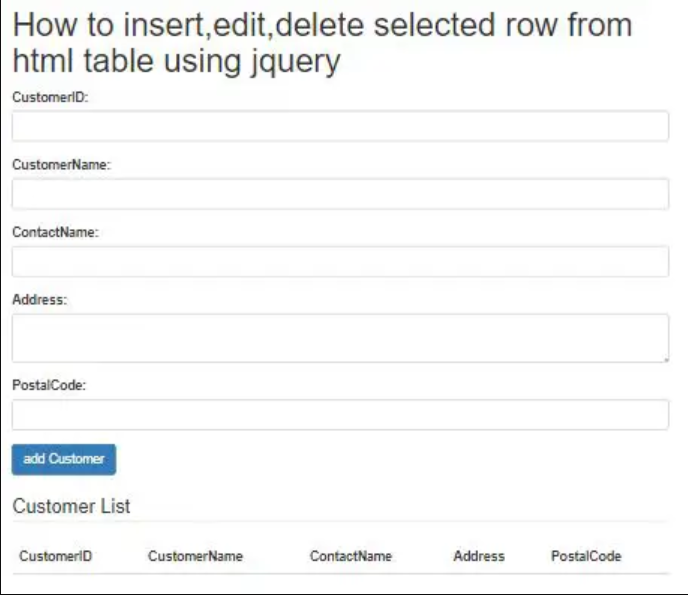
HTML
<!DOCTYPE html>
<html>
<head>
<title> Use of JQuery to Add Edit Delete Table Row </title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" />
</head>
<body>
<div class="container">
<h1> How to insert,edit,delete selected row from html table using jquery </h1>
<form id="addcustomerform">
<div class="form-group">
<label>CustomerID:</label>
<input type="text" name="txtCustomerID" id="txtCustomerID" class="form-control" value="" required="">
</div>
<div class="form-group">
<label>CustomerName:</label>
<input type="text" name="txtCustomerName" id="txtCustomerName" class="form-control" value="" required="">
</div>
<div class="form-group">
<label>ContactName:</label>
<input type="text" name="txtContactName" id="txtContactName" class="form-control" value="" required="">
</div>
<div class="form-group">
<label>Address:</label>
<textarea class="form-control" name="txtAddress" id="txtAddress"></textarea>
</div>
<div class="form-group">
<label>PostalCode:</label>
<input type="text" name="txtPostalCode" id="txtPostalCode" class="form-control" value="" required="">
</div>
<button type="submit" id="btnaddcustomer" class="btn btn-primary save-btn">add Customer</button>
</form>
<br />
<fieldset>
<legend>Customer List
</legend>
<table class="table">
<thead>
<tr>
<th>CustomerID</th>
<th>CustomerName</th>
<th>ContactName</th>
<th>Address</th>
<th>PostalCode</th>
</tr>
</thead>
<tbody id="tblbody">
</tbody>
</table>
</fieldset>
</div>
</body>
</html>
Add rows in the table using Jquery
Now let’s write the script code for adding the customer rows in the table.
<script type="text/javascript">
//add customer
$("#btnaddcustomer").on("click", function (e) {
e.preventDefault();
var CustomerID = $("#txtCustomerID").val();
var CustomerName = $("#txtCustomerName").val();
var ContactName = $("#txtContactName").val();
var Address = $("#txtAddress").val();
var PostalCode = $("#txtPostalCode").val();
var tablerow = "<tr data-CustomerID='" + CustomerID + "' data-CustomerName='" + CustomerName + "'"
+ "data-ContactName='" + ContactName + "' data-Address='" + Address + "' data-PostalCode='" + PostalCode + "'>"
+ "<td>" + CustomerID + "</td>"
+ "<td>" + CustomerName + "</td>"
+ "<td>" + ContactName + "</td>"
+ "<td>" + Address + "</td>"
+ "<td>" + PostalCode + "</td>"
+ "<td>" +
"<button class='btn btn-info btn-xs btn-editcustomer'><i class='fa fa-pencil' aria-hidden='true'></i>Edit</button>" +
"<button class='btn btn-danger btn-xs btn-deletecustomer'><i class='fa fa-trash' aria-hidden='true'>Delete</button>"
+ "</td>"
+ "</tr>";
debugger;
$("#tblbody").append(tablerow);
$("input[type='text']").each(function () {
$(this).val("");
});
$("#textarea").val('');
});
</script>
How to edit selected row from html table using jquery
Now we want to use jQuery to click on a table cell and edit the data.
On Click of the edit button, we are replacing the table cell with a text input and calls custom events so we can handle whatever use case cancel, update, delete action.
<script type="text/javascript">
//handle edit button click
$("#tblbody").on("click", ".btn-editcustomer", function () {
debugger;
var CustomerID = $(this).parents("tr").attr('data-CustomerID');
var CustomerName = $(this).parents("tr").attr('data-CustomerName');
var ContactName = $(this).parents("tr").attr('data-ContactName');
var Address = $(this).parents("tr").attr('data-Address');
var PostalCode = $(this).parents("tr").attr('data-PostalCode');
$(this).parents("tr").find("td:eq(0)").html('<input name="txtupdate_CustomerID" id="txtupdate_CustomerID" value="' + CustomerID + '">');
$(this).parents("tr").find("td:eq(1)").html('<input name="txtupdate_customerName" id="txtupdate_customerName" value="' + CustomerName + '">');
$(this).parents("tr").find("td:eq(2)").html('<input name="txtupdate_ContactName" id="txtupdate_ContactName" value="' + ContactName + '">');
$(this).parents("tr").find("td:eq(3)").html('<input name="txtupdate_Address" id="txtupdate_Address" value="' + Address + '">');
$(this).parents("tr").find("td:eq(4)").html('<input name="txtupdate_PostalCode" id="txtupdate_PostalCode" value="' + PostalCode + '">');
$(this).parents("tr").find("td:eq(5)").prepend("<button class='btn btn-primary btn-xs btn-updatecustomer'><i class='fa fa-pencil' aria-hidden='true'></i>Update</button>"
+ "<button class='btn btn-warning btn-xs btn-cancelupdate'><i class='fa fa-times' aria-hidden='true'>Cancel</button>")
$(this).hide();
});
$("#tblbody").on("click", ".btn-cancelupdate", function () {
debugger;
var CustomerID = $(this).parents("tr").attr('data-CustomerID');
var CustomerName = $(this).parents("tr").attr('data-CustomerName');
var ContactName = $(this).parents("tr").attr('data-ContactName');
var Address = $(this).parents("tr").attr('data-Address');
var PostalCode = $(this).parents("tr").attr('data-PostalCode');
$(this).parents("tr").find("td:eq(0)").text(CustomerID);
$(this).parents("tr").find("td:eq(1)").text(CustomerName);
$(this).parents("tr").find("td:eq(2)").text(ContactName);
$(this).parents("tr").find("td:eq(3)").text(Address);
$(this).parents("tr").find("td:eq(4)").text(PostalCode);
$(this).parents("tr").find(".btn-editcustomer").show();
$(this).parents("tr").find(".btn-updatecustomer").remove();
$(this).parents("tr").find(".btn-cancelupdate").remove();
});
$("#tblbody").on("click", ".btn-updatecustomer", function () {
var CustomerID = $(this).parents("tr").find("input[name='txtupdate_CustomerID']").val();
var CustomerName = $(this).parents("tr").find("input[name='txtupdate_customerName']").val();
var ContactName = $(this).parents("tr").find("input[name='txtupdate_ContactName']").val();
var Address = $(this).parents("tr").find("input[name='txtupdate_Address']").val();
var PostalCode = $(this).parents("tr").find("input[name='txtupdate_PostalCode']").val();
debugger;
$(this).parents("tr").find("td:eq(0)").text(CustomerID);
$(this).parents("tr").find("td:eq(1)").text(CustomerName);
$(this).parents("tr").find("td:eq(2)").text(ContactName);
$(this).parents("tr").find("td:eq(3)").text(Address);
$(this).parents("tr").find("td:eq(4)").text(PostalCode);
$(this).parents("tr").attr('data-CustomerID', CustomerID);
$(this).parents("tr").attr('data-CustomerName', CustomerName);
$(this).parents("tr").attr('data-ContactName', ContactName);
$(this).parents("tr").attr('data-Address', Address);
$(this).parents("tr").attr('data-PostalCode', PostalCode);
$(this).parents("tr").find(".btn-editcustomer").show();
$(this).parents("tr").find(".btn-cancelupdate").remove();
$(this).parents("tr").find(".btn-updatecustomer").remove();
});
</script>

Delete row from HTML table using Jquery
<script type="text/javascript">
//delete row from the table
$("#tblbody").on("click", ".btn-deletecustomer", function () {
$(this).parents("tr").remove();
});
</script>
Complete code for crud operations in html table using jquery
<!DOCTYPE html>
<html>
<head>
<title> Use of JQuery to Add Edit Delete Table Row </title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" />
</head>
<body>
<div class="container">
<h1> How to insert,edit,delete selected row from html table using jquery </h1>
<form id="addcustomerform">
<div class="form-group">
<label>CustomerID:</label>
<input type="text" name="txtCustomerID" id="txtCustomerID" class="form-control" value="" required="">
</div>
<div class="form-group">
<label>CustomerName:</label>
<input type="text" name="txtCustomerName" id="txtCustomerName" class="form-control" value="" required="">
</div>
<div class="form-group">
<label>ContactName:</label>
<input type="text" name="txtContactName" id="txtContactName" class="form-control" value="" required="">
</div>
<div class="form-group">
<label>Address:</label>
<textarea class="form-control" name="txtAddress" id="txtAddress"></textarea>
</div>
<div class="form-group">
<label>PostalCode:</label>
<input type="text" name="txtPostalCode" id="txtPostalCode" class="form-control" value="" required="">
</div>
<button type="submit" id="btnaddcustomer" class="btn btn-primary save-btn">add Customer</button>
</form>
<br />
<fieldset>
<legend>
Customer List
</legend>
<table class="table">
<thead>
<tr>
<th>CustomerID</th>
<th>CustomerName</th>
<th>ContactName</th>
<th>Address</th>
<th>PostalCode</th>
</tr>
</thead>
<tbody id="tblbody"></tbody>
</table>
</fieldset>
</div>
</body>
</html>
<script type="text/javascript">
//add customer
$("#btnaddcustomer").on("click", function (e) {
e.preventDefault();
var CustomerID = $("#txtCustomerID").val();
var CustomerName = $("#txtCustomerName").val();
var ContactName = $("#txtContactName").val();
var Address = $("#txtAddress").val();
var PostalCode = $("#txtPostalCode").val();
var tablerow = "<tr data-CustomerID='" + CustomerID + "' data-CustomerName='" + CustomerName + "'"
+ "data-ContactName='" + ContactName + "' data-Address='" + Address + "' data-PostalCode='" + PostalCode + "'>"
+ "<td>" + CustomerID + "</td>"
+ "<td>" + CustomerName + "</td>"
+ "<td>" + ContactName + "</td>"
+ "<td>" + Address + "</td>"
+ "<td>" + PostalCode + "</td>"
+ "<td>" +
"<button class='btn btn-info btn-xs btn-editcustomer'><i class='fa fa-pencil' aria-hidden='true'></i>Edit</button>" +
"<button class='btn btn-danger btn-xs btn-deletecustomer'><i class='fa fa-trash' aria-hidden='true'>Delete</button>"
+ "</td>"
+ "</tr>";
debugger;
$("#tblbody").append(tablerow);
$("input[type='text']").each(function () {
$(this).val("");
});
$("#textarea").val('');
});
</script>
<script type="text/javascript">
//handle edit button click
$("#tblbody").on("click", ".btn-editcustomer", function () {
debugger;
var CustomerID = $(this).parents("tr").attr('data-CustomerID');
var CustomerName = $(this).parents("tr").attr('data-CustomerName');
var ContactName = $(this).parents("tr").attr('data-ContactName');
var Address = $(this).parents("tr").attr('data-Address');
var PostalCode = $(this).parents("tr").attr('data-PostalCode');
$(this).parents("tr").find("td:eq(0)").html('<input name="txtupdate_CustomerID" id="txtupdate_CustomerID" value="' + CustomerID + '">');
$(this).parents("tr").find("td:eq(1)").html('<input name="txtupdate_customerName" id="txtupdate_customerName" value="' + CustomerName + '">');
$(this).parents("tr").find("td:eq(2)").html('<input name="txtupdate_ContactName" id="txtupdate_ContactName" value="' + ContactName + '">');
$(this).parents("tr").find("td:eq(3)").html('<input name="txtupdate_Address" id="txtupdate_Address" value="' + Address + '">');
$(this).parents("tr").find("td:eq(4)").html('<input name="txtupdate_PostalCode" id="txtupdate_PostalCode" value="' + PostalCode + '">');
$(this).parents("tr").find("td:eq(5)").prepend("<button class='btn btn-primary btn-xs btn-updatecustomer'><i class='fa fa-pencil' aria-hidden='true'></i>Update</button>"
+ "<button class='btn btn-warning btn-xs btn-cancelupdate'><i class='fa fa-times' aria-hidden='true'>Cancel</button>")
$(this).hide();
});
$("#tblbody").on("click", ".btn-cancelupdate", function () {
debugger;
var CustomerID = $(this).parents("tr").attr('data-CustomerID');
var CustomerName = $(this).parents("tr").attr('data-CustomerName');
var ContactName = $(this).parents("tr").attr('data-ContactName');
var Address = $(this).parents("tr").attr('data-Address');
var PostalCode = $(this).parents("tr").attr('data-PostalCode');
$(this).parents("tr").find("td:eq(0)").text(CustomerID);
$(this).parents("tr").find("td:eq(1)").text(CustomerName);
$(this).parents("tr").find("td:eq(2)").text(ContactName);
$(this).parents("tr").find("td:eq(3)").text(Address);
$(this).parents("tr").find("td:eq(4)").text(PostalCode);
$(this).parents("tr").find(".btn-editcustomer").show();
$(this).parents("tr").find(".btn-updatecustomer").remove();
$(this).parents("tr").find(".btn-cancelupdate").remove();
});
$("#tblbody").on("click", ".btn-updatecustomer", function () {
var CustomerID = $(this).parents("tr").find("input[name='txtupdate_CustomerID']").val();
var CustomerName = $(this).parents("tr").find("input[name='txtupdate_customerName']").val();
var ContactName = $(this).parents("tr").find("input[name='txtupdate_ContactName']").val();
var Address = $(this).parents("tr").find("input[name='txtupdate_Address']").val();
var PostalCode = $(this).parents("tr").find("input[name='txtupdate_PostalCode']").val();
debugger;
$(this).parents("tr").find("td:eq(0)").text(CustomerID);
$(this).parents("tr").find("td:eq(1)").text(CustomerName);
$(this).parents("tr").find("td:eq(2)").text(ContactName);
$(this).parents("tr").find("td:eq(3)").text(Address);
$(this).parents("tr").find("td:eq(4)").text(PostalCode);
$(this).parents("tr").attr('data-CustomerID', CustomerID);
$(this).parents("tr").attr('data-CustomerName', CustomerName);
$(this).parents("tr").attr('data-ContactName', ContactName);
$(this).parents("tr").attr('data-Address', Address);
$(this).parents("tr").attr('data-PostalCode', PostalCode);
$(this).parents("tr").find(".btn-editcustomer").show();
$(this).parents("tr").find(".btn-cancelupdate").remove();
$(this).parents("tr").find(".btn-updatecustomer").remove();
});
</script>
<script type="text/javascript">
//delete row from the table
$("#tblbody").on("click", ".btn-deletecustomer", function () {
$(this).parents("tr").remove();
});
</script>
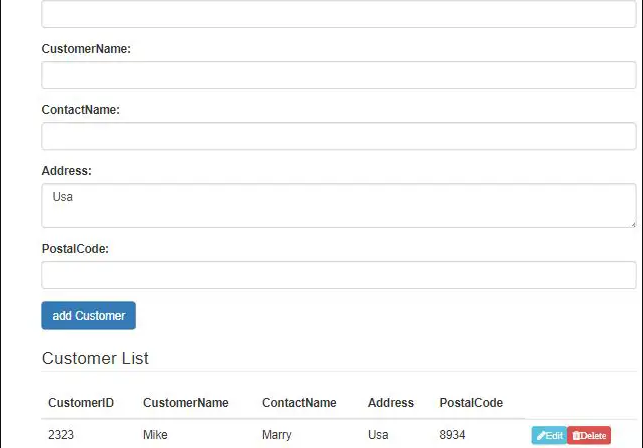