[BEST WAY]-HOW TO DISPLAY JSON DATA IN HTML USING AJAX
DISPLAY JSON DATA IN HTML USING AJAX
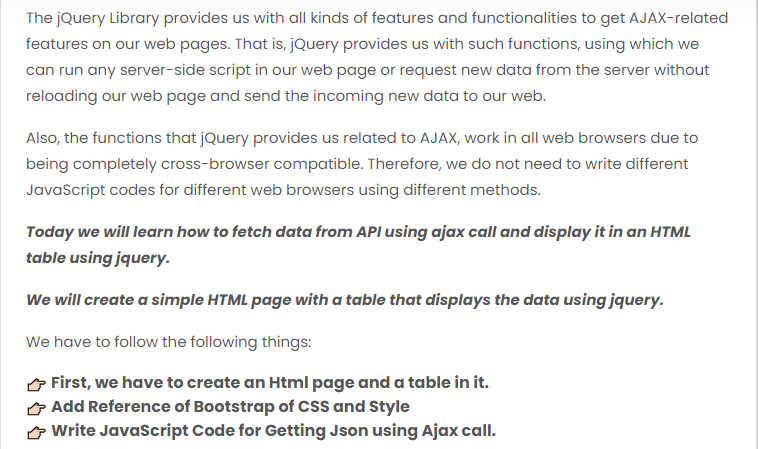

Step 1: Create Html page and table
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
</head>
<body>
<div class="container">
<h1>How to display JSON data in HTML using ajax</h1>
<br />
<table class="table">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Address</th>
<th>City</th>
<th>ZipCode</th>
</tr>
</thead>
<tbody id="tblbody"></tbody>
</table>
</div>
</body>
</html>
Step 2: Display JSON data from URL using Ajax
For Our understanding purpose, let take an example, I have an API that returns the list of employees in the organization.
Now let’s say we want to bind that JSON response in the HTML table. Basically, we are going I want to Display JSON data in an HTML table using jQuery.
Json Response:
[
{
"$id": "1",
"Id": 1,
"Name": "Pascale Cartrain",
"Address": "Rua do Mercado, 12",
"City": "Walla",
"ZipCode": "243232"
},
{
"$id": "2",
"Id": 2,
"Name": "Liu Wong",
"Address": "DaVinci",
"City": "Paris",
"ZipCode": "243232"
},
{
"$id": "3",
"Id": 3,
"Name": "Miguel",
"Address": "Avda. Azteca",
"City": "London",
"ZipCode": "243232"
},
{
"$id": "4",
"Id": 4,
"Name": "Anabela",
"Address": "ul. Filtrowa 68",
"City": "New Delhi",
"ZipCode": "243232"
},
{
"$id": "5",
"Id": 5,
"Name": "Mary Saveley",
"Address": "Adenauerallee",
"City": "Tokyo",
"ZipCode": "243232"
}
]
Display JSON response from Ajax as HTML or table
Here I’m calling the ajax function and displaying the JSON data, to a table which easy to read by the user. On success of Ajax call function Bind Data with Jquery Each() javascript() which loop through each object in JSON array,.
<script type="text/javascript">
$(document).ready(function () {
GetBindData()
});
//Get all employees
function GetBindData() {
$.ajax({
url: 'http://restapi.adequateshop.com/api/Metadata/GetEmployees',
method: 'GET',
dataType: 'json',
contentType: 'application/json; charset=utf-8',
success: function (result)
{
//after successfully call bind data to Table
BindDataWithJqueyEach(result);
},
fail: function (jqXHR, textStatus) {
alert("Request failed: " + textStatus);
}
})
}
function BindDataToTable(data)
{
if (data != null && data) {
for (var i = 0; i < data.length; i++) {
var tablerow = "<tr>"
+ "<td>" + data[i].Id + "</td>"
+ "<td>" + data[i].Name + "</td>"
+ "<td>" + data[i].Address + "</td>"
+ "<td>" + data[i].City + "</td>"
+ "<td>" + data[i].ZipCode + "</td>"
+ "</tr>";
$("#tblbody").append(tablerow);
}
}
}
</script>
Method-2 Using jQuery.each function bind data in the table

function BindDataWithJqueyEach(data)
{
jQuery.each(data, function (i, val) {
var tablerow = "<tr>"
+ "<td>" + val.Id + "</td>"
+ "<td>" + val.Name + "</td>"
+ "<td>" + val.Address + "</td>"
+ "<td>" + val.City + "</td>"
+ "<td>" + val.ZipCode + "</td>"
+ "</tr>";
$("#tblbody").append(tablerow);
});
}
Full Code
function GetBindemployeesData() {
$.ajax({
url: 'http://restapi.adequateshop.com/api/Metadata/GetEmployees',
method: 'GET',
dataType: 'json',
contentType: 'application/json; charset=utf-8',
success: function (result) {
//after successfully call bind data to Table
if (result && result.length > 0)
{
jQuery.each(result, function (i, val) {
var tablerow = "<tr>"
+ "<td>" + val.Id + "</td>"
+ "<td>" + val.Name + "</td>"
+ "<td>" + val.Address + "</td>"
+ "<td>" + val.City + "</td>"
+ "<td>" + val.ZipCode + "</td>"
+ "</tr>";
$("#tblbody").append(tablerow);
});
}
},
fail: function (jqXHR, textStatus) {
alert("Request failed: " + textStatus);
}
})
}
Output:-
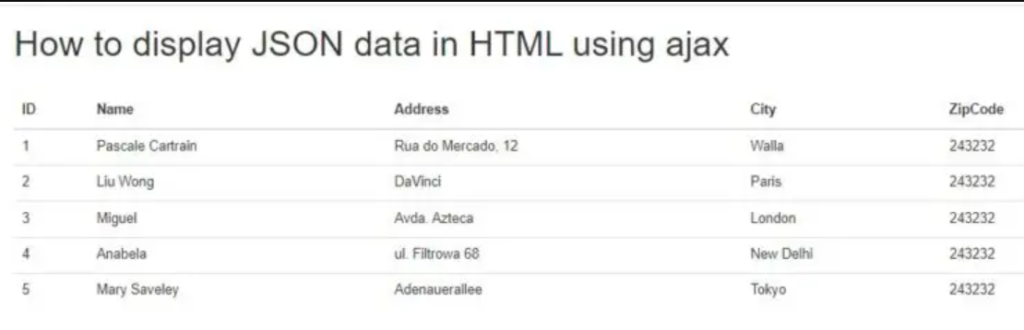